It’s very common for software developers to lose hours while trying to decipher the purpose of a piece of code. Developers spend far more time reading code than actually writing it. The renowned Robert Martin, often referred to as Uncle Bob, revealed a significant 10:1 ratio between reading and coding. This implies that unless we comprehend the code we're reviewing, our ability to produce functional code diminishes.
We all want to finish our work quickly and move on, but failing to revisit and improve our code before declaring a task finished might provide significant difficulties later on when attempting to understand the functionality of the code. If the code is messy, even the most skilled teams may face a slowdown. The number of team members working on a project loses importance because the codebase just gets slower. New developers will spend a significant amount of time trying to understand the project.
It makes sense that our code won't be perfectly clean right away. The way the human mind works is chaotic, switching from high-level to low-level reasoning frequently within a single line. Making the code functional first should be our top focus, but we should be prepared for some pointless code to appear and yet keep the system running. We must, however, stop ourselves from leaving it in that condition. Before a task may be considered finished, it must be corrected.
In this article, we're going to talk about clean code, explore its essential best practices while taking a more detailed look of the SOLID principles.
What Is Clean Code?
As programmers, we are constantly faced with the challenge of writing code that is simple to read, maintain, and modify. Here, the idea of "Clean Coding" is put into use. Clean code is a term frequently mentioned among professional software developers in discussions about the quality of their work. While it might be tempting to view code that simply accomplishes its task as good, the best programmers understand that quality code goes beyond functionality. They emphasize that code should not only function correctly but should also adhere to principles of readability, maintainability, and performance. By adhering to clean code practices, your code becomes more comprehensible, adaptable, and troubleshoot-friendly. Additionally, it gains efficiency and a focused structure.
Furthermore, it is crucial in reducing technical debt, which is the cost associated with maintaining a codebase over time. A codebase that is simple to understand and maintain not only saves time and money over time but also makes it easier for developers to work together.
Essentially, elegant, optimized, maintainable, concise, and ready for future improvements are characteristics of clean code. This combination of qualities results in code that is enjoyable to work with. It's crucial to remember that writing clean code is not an easy task. It necessitates adherence to a number of rules and ideas that direct your coding procedures in the right way.
Clean Code Best Practices
Let’s check out some key practices for clean code. Implementing these practices, you foster a codebase that is easy to understand, maintain, and expand, resulting in a more efficient and collaborative development environment.
Meaningful Naming
Choose descriptive and self-explanatory names for variables, functions, and classes. Avoid using cryptic or ambiguous names that require readers to decipher their purpose. This reduces the need for mental mapping and enhances code comprehension. Aim for simplicity in naming by using a single word to represent each unique concept. This approach makes it easier to grasp the purpose of different elements in your codebase.
Concise Functions
Craft functions that are concise, well-defined, and focused on performing a single task. This approach improves code readability and helps in maintaining a modular codebase. Avoid duplicating code fragments. Instead, centralize shared logic or data into reusable components, reducing redundancy and making maintenance more efficient. Organize your code into logical structures that reflect its purpose. Keep functions and classes focused on specific responsibilities, which aids in comprehending and maintaining the codebase.
Effective Comments
Use comments to clarify complex logic, assumptions, or business rules that might not be evident from the code alone. However, avoid over-commenting, and prioritize comments that provide valuable insights.
Consistent Code Formatting
Adhere to a consistent coding style, including indentation, spacing, and naming conventions. Consistency in formatting enhances collaboration and makes the codebase more professional.
Minimal Class Complexity
Create classes that have a clear and single responsibility. Avoid incorporating excessive functionalities within a single class, as it can lead to confusion and challenges in maintaining the code. When creating abstractions, clearly define their roles and responsibilities. Isolate them from unnecessary details and ensure they interact effectively with other components.
Error Handling
Handle errors gracefully by incorporating error-checking mechanisms. Establish a standard flow for error management and use try-catch-finally blocks for checked exceptions. For unchecked exceptions, provide informative error messages to aid debugging.
Thorough Testing
Write unit tests to validate individual units of code functionality. Additionally, consider integration tests that examine interactions between components and end-to-end tests that simulate real-world scenarios. Implement safety measures like code reviews, continuous integration, and automated testing. These practices help catch issues early in the development process, ensuring a higher level of code quality.
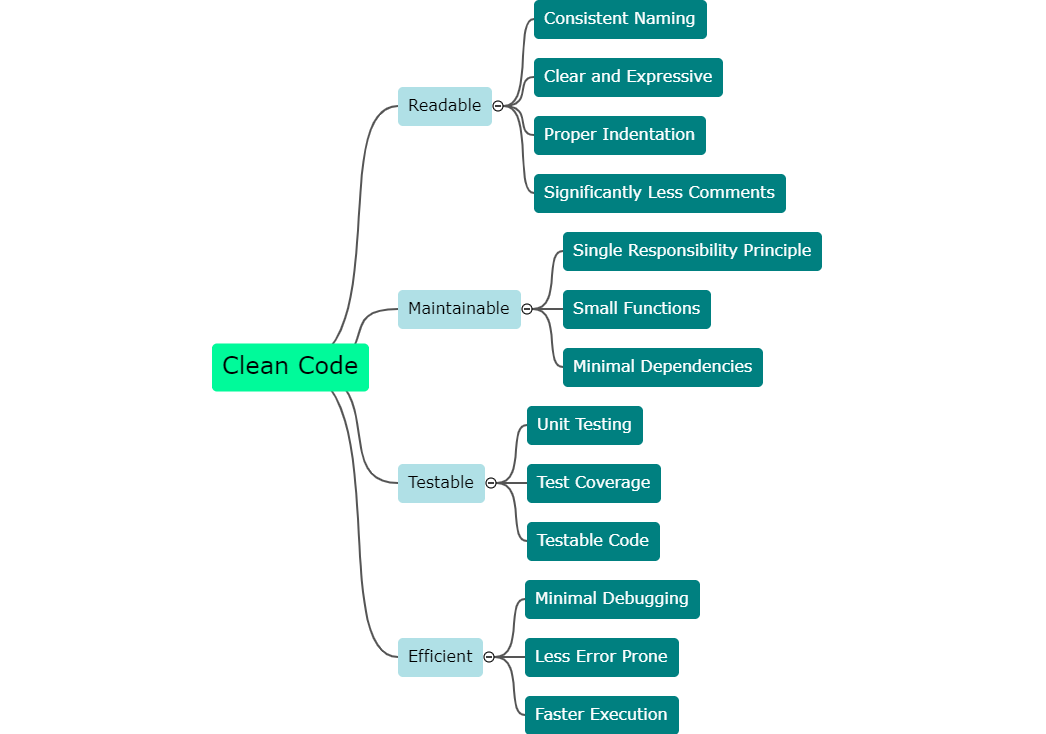
A Deep Dive into SOLID Principles
Now that we've covered some broad best practices for writing clean code, let's dig deeper into one of the most widely used programming design principles. This principle is critical to the successful implementation of the majority of the aforementioned practices.
By encapsulating the core philosophies of coding, design principles serve as compass points that guide you towards becoming a more capable programmer. Among the many principles available, we will concentrate on five essential ones, conveniently summarized by the acronym SOLID.
Single Responsibility Principle
The "S" in SOLID stands for Single Responsibility. This principle teaches us to break down our code into modules, each with a single responsibility. When faced with a large class performing unrelated tasks, it's advisable to divide it into separate classes. This approach not only increases code volume but also enables clear identification of each class's purpose. It facilitates cleaner testing and allows for reusable components without the baggage of irrelevant methods. This becomes increasingly crucial as we advance.
# Single Responsibility Principle (SRP)
# Each class should have a single responsibility.
class Shape(ABC):
@abstractmethod
def calculate_area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def calculate_area(self):
return math.pi * self.radius ** 2
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def calculate_area(self):
return self.width * self.height
Open-Closed Principle
The Open-Closed principle encourages designing modules in a way that permits adding new functionality without modifying existing code. Instead of altering modules directly, we should extend them through methods like wrapping. This practice ensures that once a module is in use, it remains locked, reducing the risk of new additions breaking the code.
# Open/Closed Principle (OCP)
# Software entities should be open for extension but closed for modification.
class AreaCalculator:
def calculate(self, shape):
if isinstance(shape, Shape):
return shape.calculate_area()
else:
raise ValueError("Invalid shape provided")
Liskov Substitution Principle
The Liskov Substitution principle guides us in extending legacy code cautiously. This principle suggests that we should only extend modules when we're certain that the extension maintains the core essence of the module.
# Liskov Substitution Principle (LSP)
# Subtypes must be substitutable for their base types.
def print_area(area_calculator, shape):
if isinstance(shape, Shape):
area = area_calculator.calculate(shape)
print(f"The area is: {area}")
else:
print("Invalid shape provided")
Interface Segregation Principle
Moving on, we encounter "I" for Interface Segregation. This principle advocates splitting modules into smaller abstractions, such as interfaces, that encapsulate the exact functionality required by the module. This approach proves especially beneficial for testing as it enables mocking only the necessary functionality for each module.
# Interface Segregation Principle (ISP)
# Clients should not be forced to depend on interfaces they do not use.
class PrintableShape(ABC):
@abstractmethod
def print_shape(self):
pass
class CirclePrintable(Circle, PrintableShape):
def print_shape(self):
print("Printing Circle")
Dependency Inversion Principle
Finally, we have "D" for Dependency Inversion. This principle promotes abstract communication between parts of the code. Rather than direct interactions, modules should communicate abstractly, usually via defined interfaces. This technique eliminates direct relationships between code segments, enhancing module isolation and enabling parts to be swapped out as needed.
Combining these SOLID principles results in decoupled code, yielding independent modules. This, in turn, enhances code maintainability, scalability, reusability, and testability.
# Dependency Inversion Principle (DIP)
# High-level modules should not depend on low-level modules. Both should depend on abstractions.
class AreaCalculatorClient:
def __init__(self, area_calculator):
self.area_calculator = area_calculator
def calculate_and_print(self, shape):
if isinstance(shape, Shape):
area = self.area_calculator.calculate(shape)
print(f"The area is: {area}")
else:
print("Invalid shape provided")
Conclusion
Clean code is a great way to improve a project's readability and maintainability. Furthermore, it encourages developer collaboration, reduces debugging time, and paves the way for efficient scaling and adaptation. Developers can create a codebase that demonstrates professionalism and expertise by following principles such as meaningful naming, effective commenting, consistent code formatting, etc. Adopting clean code practices not only improves the quality of the software produced, but it also lays the groundwork for a more efficient development process and a more sustainable technological future. Commitment to writing clean code remains an essential cornerstone for building robust, adaptable, and successful software solutions as the digital landscape evolves.
The Expertise of Solwey Consulting
At Solwey Consulting, we specialize in custom software development services, offering top-notch solutions to help businesses like yours achieve their growth objectives. With a deep understanding of technology, our team of experts excels in identifying and using the most effective tools for your needs, making us one of the top custom software development companies in Austin, TX.
Whether you need ecommerce development services or custom software consulting, our custom-tailored software solutions are designed to address your unique requirements. We are dedicated to providing you with the guidance and support you need to succeed in today's competitive marketplace.
If you have any questions about our services or are interested in learning more about how we can assist your business, we invite you to reach out to us. At Solwey Consulting, we are committed to helping you thrive in the digital landscape.